Pre-Requisites
Step 1: Create a New Folder
Navigate to desired drive on your computer and create a new folder for Playwright (ex: PlaywrightFramework).
Step 2: Open the newly created folder in Visual Studio Code ID
From Visual Studio Code, Navigate to File> Open > Choose the newly created folder (PlaywrightFramework).
Step 3: Create a package.json file
The package.json file helps to keep track of all node modules installed and it also helps to create shortcuts to run Playwright tests.
From your Visual Studio Code > Open Terminal and execute the below command.
npm init
Once you execute the above command it asks you a set of questions just answer them or Press Enter until package.json gets created.
Step 4: Install Playwright Tests npm package
The playwright has its own test runner for end-to-end tests, we call it the Playwright Test.
From your Visual Studio Code > Open Terminal and execute the below command.
npm i -D @playwright/test

Step 5: Install the Playwright npm package
Unlike Playwright Library, Playwright Test does not bundle browsers by default, so you need to install them explicitly.
From your Visual Studio Code > Open Terminal and execute the below command.
npm install --save-dev playwright
Step 6: Create an src Folder inside your Project Folder
Src folder holds all your tests and page objects, it helps us to manage tests in an easy way.
Create New Folder called src inside Project Folder (Ex: PlaywrightFramework).
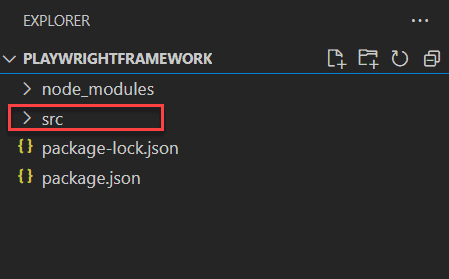
Step 7: Create Folders for Tests and Page Object
Inside your src folder created in the above step create two more folders namely specs and pages.
Your directory structure should look like the below.
PlaywrightFramework
-src
--tests
--pages
tests folder contains all your Playwright test files
pages folder contains all your Playwright page object files
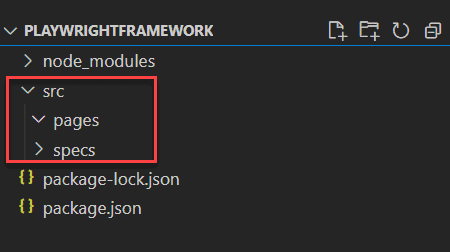
Step 8: Create First Page Object File with Playwright
Inside your pages folder create a file name it as example.page.ts
In this tutorial, we are going to write two simple test cases.
- Navigate to google.com and Verify
- Search for the keyword Playwright and verify search results
Copy and Paste the below code into your newly created file example.page.ts
//example.page.ts
import type { Page } from '@playwright/test';
export class ExampleClass{
readonly page: Page
constructor(page:Page){
this.page=page
}
async typeSearchText(){
await this.page.type('inpute="q"]',"Playwright")
}
async pressEnter(){
await this.page.keyboard.press('Enter
}
async searchResult(){
return this.page.innerText('//h3tains(text(),"Playwright:")]')
}
}
Step 9: Create First Test /Spec File with Playwright
Inside your pages folder create a file name it as example.spec.ts
The example.spec.ts contains your actual test script or specs which you create using Playwright libraries.
Copy and Paste the below code into your newly created example.spec.ts
//example.spec.ts
import { test, expect } from '@playwright/test';
import { ExampleClass } from '../pages/example.page';test('Navigatele', async ({ page }) => {
await page.goto('https://google.com/');
const url = await page.url();
expect(url).toContain('google');
});test('Search for Playwright', async ({ page }) => {
await page.goto('https://google.com/');
let exampletest = new ExampleClass(page);
await exampletest.typeSearchText();
await exampletest.pressEnter();
const text = await exampletest.searchResult();
await console.log(text);
expect(text).toContain('Playwright: Fast and reliable');
});
Step 10: Execute or Run your First Test with a Playwright
The playwright provides options to execute your test in both headed mode and headless mode. Let’s try headed mode execution with the chrome browser.
From your Visual Studio Code Terminal Execute the below command.
npx playwright test --headed
Once your tests are executed Playwright shows the results in the command line or Visual Studio Terminal.
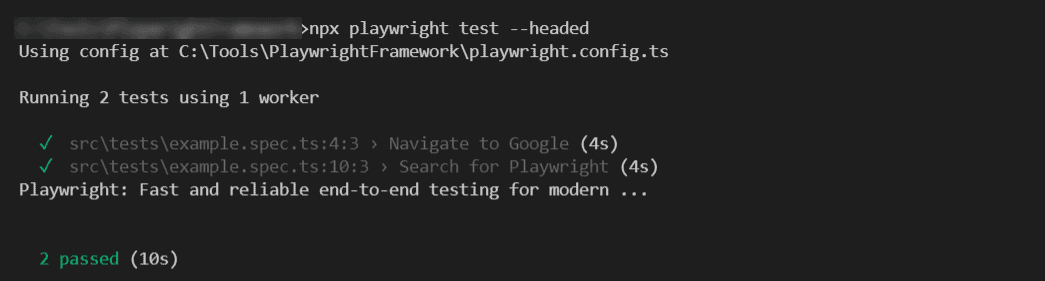
Configure PlaywrightFramework behavior with Playwright Global configuration File
The playwright provides the option to create the configuration file, where you can specify values such as browser name, baseUrl, width, and height.
Create a playwright.config.ts file in your Project Folder (root level)
From your Visual Studio Code, Create a new file name as playwright.config.ts, This file must be created inside your Project Folder (ex: PlaywrightFramework) at the root level
The sample configuration file for Playwright looks like below.
//playwright.config.ts
import { PlaywrightTestConfig } from '@playwright/test';
const config: PlaywrightTestConfig = {
use: {
baseURL: 'http://google.com/',
browserName: 'chrome',
headless: false,
},
};
export default config;
The above is a sample file there are many options that Playwright configuration supports. Read the details here.
Playwright Supported Reporters
Currently, Playwright Supports Reporters
- Dot Reporter
- Line Reporter
- JUnit Reporter
- JSON Reporter
- List Reporter
Playwright allows us to configure multiple reporters as well, Playwright reporters can be mentioned via command line while execution test, or we can set them in the global configuration file.
Example of the global configuration file for Playwright Reporter:
// playwright.config.ts
import { PlaywrightTestConfig } from '@playwright/test';const config: PlaywrightTestConfig = {
reporter: [ ['junit', { outputFile: 'results.xml' }] ],
};
export default config;
Generating HTML Reporter with Playwright Test Automation Tool
The playwright doesn’t support HTML reports for tests by default, but it supports JUnit XML files, However, using JUnit reporter and third-party npm packages such as XUnit Viewer we can generate the HTML reporter.
Playwright supports allure report as well but as of now, it’s an experimental feature.